Image
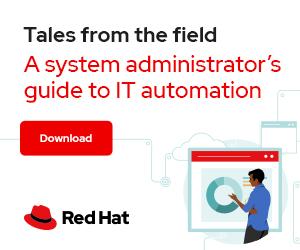
Sysadmins modify lots of files. Sometimes they're code. Other times they're configuration files, YAML playbooks, XML, policy documents, kickstart files, and probably a few takeaway lunch orders, too. It's important to track what you've changed and share your changes with others who may need to adjust their local copies of files.
To make this process seamless, many online Git repository providers have adopted the "merge request" or "pull request" model, in which they expect contributors to clone (often called "fork," even though the intent is actually not to fork the project) the entire repository and then submit a request through the online platform to integrate the changed branch back into the original repo. But what do you do when you're not using a Git platform-as-a-service (PaaS) provider or when you want a streamlined process for submitting changes?
You use diff
and patch
. These are the tools everyone used before online Git hosts moved the process into the browser, and they're just as valid today as ever. With the git diff
command, you can create a record of how the file has changed, and the owner of a repository can use the patch
command to "replay" those changes over the old version to bring it up to date with the new version.
Suppose you and I are collaborating on a project to calculate prime numbers. I'll use an example written in Lua because it's easy to read. You don't actually need to know Lua for this example to be relevant. So far, the file prime.lua
contains:
function prime(num)
if num%2 == 0 then
return false
end
return true
end
It's valid code, it works as expected, but it's incomplete. So you send the file to me, and I save it as prime_revision.lua
and make some changes to it. I change some of your existing code, and I add some of my own:
function prime(num)
if num<2 or num%2 == 0 and not num == 2 then
return false
end
return true
end
num=0
while ( num >= 0 ) do
if prime(num) then
print(num .. " is prime")
end
num=num+1
end
With a small file such as this, it's relatively trivial to see what's changed. The if
statement was updated, and a bunch of code was added at the end. However, with larger files, updating the original file to match the new one would be difficult and prone to mistakes. The git diff
and patch
commands solve this problem.
When you change a committed file in Git, you can see the changes you've made using git diff
:
$ git diff prime.lua
diff --git a/prime.lua b/prime.lua
index b4c3d7c..6dea598 100644
--- a/prime.lua
+++ b/prime.lua
@@ -1,6 +1,15 @@
function prime(num)
- if num%2 == 0 then
+ if num<2 or num%2 == 0 and not num == 2 then
return false
end
return true
end
+
+num=0
+
+while ( num >= 0 ) do
+ if prime(num) then
+ print(num .. " is prime")
+ end
+ num=num+1
+end
This is the same view as the one provided by the diff
command using the --unified
option, and it's also the correct syntax to use with the patch
command. A plus sign (+
) at the beginning of a line indicates something added to the old file. A minus sign (-
) at the beginning of a line indicates a line that was removed or changed.
The git diff
command output is a valid patch file, in addition to being informative to the Git repo owner. You can do this using standard Bash redirection:
$ git diff prime.lua > prime.patch
The contents of the file are exactly the same as what was output to the terminal.
[ Download the free Bash shell scripting cheat sheet to keep the basics close at hand. ]
Once I have a patch file containing all of my changes, I can send it to you to review and, optionally, apply to your old file. You apply a patch with the patch
command:
$ patch prime.lua prime.patch
Lines were added, lines were subtracted, and in the end, you end up with a file identical to my version:
$ cat prime.lua
function prime(num)
if num<2 or num%2 == 0 and not num == 2 then
return false
end
return true
end
num=0
while ( num >= 0 ) do
if prime(num) then
print(num .. " is prime")
end
num=num+1
end
Now you can add and commit the new version, and the "merge request" is satisfied, with no external Git hosting service in sight!
There's no limit to how many times you can patch a file. You can iterate on changes, generate new patches, and send them to collaborators and maintainers. Sending changes rather than final results lets each contributor review what's changed, decide what they want to accept or decline, and apply the changes easily.
Seth Kenlon is a UNIX geek and free software enthusiast. More about me