Image
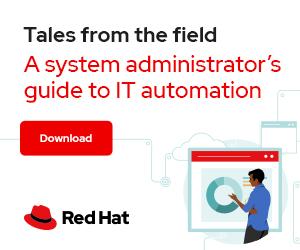
Photo by Vlada Karpovich from Pexels
It's an old quote, but I think this principle from Perl inventor Larry Wall is still valid:
"The lazy programmer will reuse whatever they can to get their work done. This virtue leads to code reuse by encouraging code to be written only once."
— Larry Wall, "The three virtues of a programmer"
Let's say you get tired of typing the same sequence of commands and finally create a nice shell script to run things with fewer keystrokes. But sometimes you want to run it with different arguments, and you definitely do not want to duplicate your great script. You need a way to provide and use arguments from the command line. This article covers exactly that.
Shell scripts have access to some "magic" variables from the environment:
$0
- The name of the script$1
- The first argument sent to the script$2
- The second argument sent to the script$3
- The third argument... and so forth$#
- The number of arguments provided$@
- A list of all arguments provided
#!/bin/bash
if [ $# -eq 0 ];
then
echo "$0: Missing arguments"
exit 1
elif [ $# -gt 2 ];
then
echo "$0: Too many arguments: $@"
exit 1
else
echo "We got some argument(s)"
echo "==========================="
echo "Number of arguments.: $#"
echo "List of arguments...: $@"
echo "Arg #1..............: $1"
echo "Arg #2..............: $2"
echo "==========================="
fi
echo "And then we do something with $1 $2"
Here are a few examples of executing this with different combinations of arguments:
$ ./ex01.sh
./ex01.sh: Missing arguments
$ ./ex01.sh aa
We got some argument(s)
===========================
Number of arguments.: 1
List of arguments...: aa
Arg #1..............: aa
Arg #2..............:
===========================
And then we do something with aa
$ ./ex01.sh aa bb
We got some argument(s)
===========================
Number of arguments.: 2
List of arguments...: aa bb
Arg #1..............: aa
Arg #2..............: bb
===========================
And then we do something with aa bb
$ ./ex01.sh aa bb cc
./ex01.sh: Too many arguments: aa bb cc
This is fine if you have one or two arguments. However, notice the default separator between arguments is the Space character. And because Space is the default separator for arguments, interesting things can happen. For example, this is what happens if you use quotes with more than two arguments:
$ ./ex01.sh aa "bb cc xx yy zz"
We got some argument(s)
===========================
Number of arguments.: 2
List of arguments...: aa bb cc xx yy zz
Arg #1..............: aa
Arg #2..............: bb cc xx yy zz
===========================
And then we do something with aa bb cc xx yy zz
Notice that the script interprets bb cc xx yy zz
as one argument because the values are enclosed in double quotes, which is also fine if this is what you want.
[ Keep your Linux commands close at hand. Download the Advanced Linux commands cheat sheet. ]
Another interesting thing is when your script gets arguments from variables. If the variable contains spaces, you need to decide if you want that value to be treated as a single argument or all parts to be treated as different arguments:
$ export AUTHOR="John Lennon"
$ export SONG="Imagine"
$ ./ex01.sh $AUTHOR $SONG
./ex01.sh: Too many arguments: John Lennon Imagine
$ ./ex01.sh "$AUTHOR" "$SONG"
We got some argument(s)
===========================
Number of arguments.: 2
List of arguments...: John Lennon Imagine
Arg #1..............: John Lennon
Arg #2..............: Imagine
===========================
And then we do something with John Lennon Imagine
When you send the arguments as unquoted variables, the script interprets all of them as separate words and "sees" three arguments: John, Lennon, and Imagine.
Using quotes around the variables made the script receive each variable as one argument, which makes more sense in this case.
As you saw, the magic variable $@
contains the list of all arguments received by the script. You can use a loop to process all the arguments:
#!/bin/bash
echo "We received $# argument(s)"
for i in "$@"
do
echo "Arg...: $i"
done
Which gives you this:
$ ./ex02.sh a b c e "stuff with spaces"
We received 5 argument(s)
Arg...: a
Arg...: b
Arg...: c
Arg...: e
Arg...: stuff with spaces
The important things when dealing with arguments in your shell scripts are to have a clear understanding of:
I hope this helps you continue being lazy in the right way... by improving or reusing some of your scripts.
Roberto Nozaki (RHCSA/RHCE/RHCA) is an Automation Principal Consultant at Red Hat Canada where he specializes in IT automation with Ansible. More about me